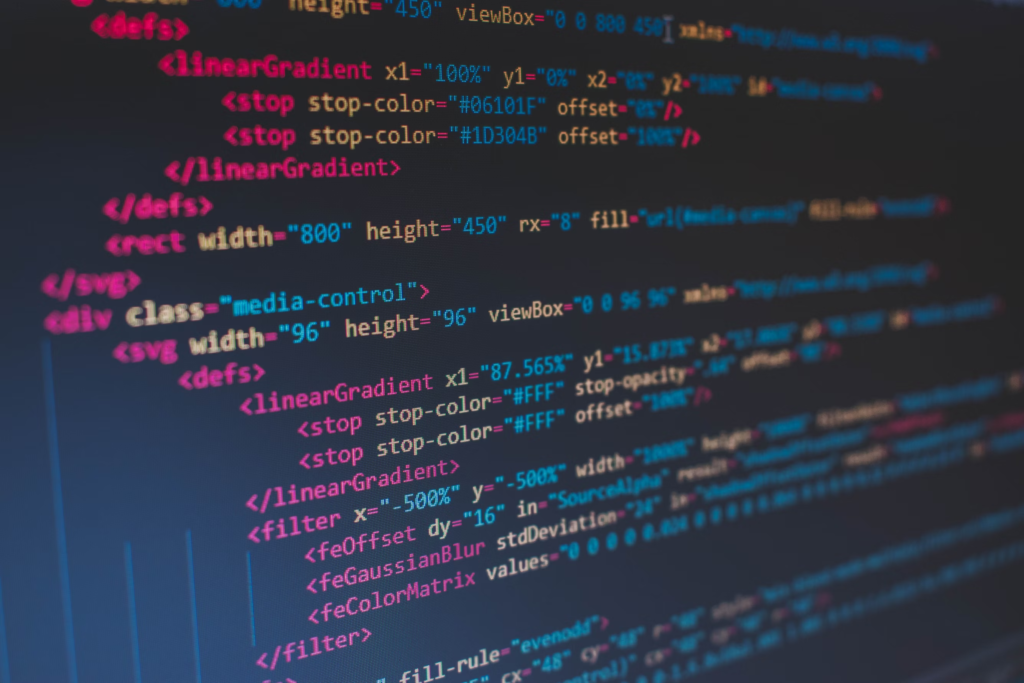
Today, I’ll dive into Pseudo Code, a simplified language that allows us to plan our software or application before we start coding. Whether you’re a new developer or a seasoned programmer, understanding the significance and application of pseudo code is invaluable.
Pseudo code isn’t just for rookies; it’s also a great way for experienced developers to sketch out their ideas, collaborate and communicate their algorithms to team members not fluent in a specific programming language.
Understanding Pseudo Code
Pseudo code, as the name suggests, isn’t an actual programming language. Instead, it’s a high-level description of a computer program or algorithm. It uses plain language mixed with some syntax of a regular programming language, making it easier to understand for anyone, regardless of their technical skills.
The Purpose of Pseudo Code
Pseudo code serves as a kind of draft blueprint for your final program. It allows developers to think through their algorithm’s logic without getting confused or lost in the nitty-gritty of specific syntax. Once the pseudo code is in place, it’s much easier to translate the logic into real, executable code.
The Purpose of Pseudo Code
Programming has three main control structures: Sequence, Selection, and Repetition. Let’s take a closer look at these individually.
1. Sequence
This is the most straightforward control structure where instructions are executed one after another. For example, let’s consider a day in your life. Wake up, brush teeth, have breakfast, and finally start your laptop for work. This routine executes in the exact order every day, much like a sequence in pseudocode.
Start
Wake up
Brush teeth
Have breakfast
Start laptop
End
2. Selection
Selection in pseudocode allows the execution or skipping of commands based on a true/false condition. Think of it as an ‘If-Else’ statement. For instance, an analog alarm clock. ‘If’ it’s 6 AM, the alarm rings. ‘Else’, it doesn’t.
Start
If Current Time = 6 AM Then
Ring Alarm
Else
Don't Ring Alarm
End If
End
3. Repetition
This structure repeats commands until a condition evaluates to true or false. These loops are useful when you have to perform a task multiple times. Imagine your weekly grocery shopping routine. ‘While’ there are items in your shopping list, keep adding them to your cart.
Start
While there are items in the shopping list
Add item to the shopping cart
End While
End
Furthermore, pseudocode often involves operators such as addition(+), subtraction(-), multiplication(*), and division(/). Boolean operators like ‘AND’, ‘OR’, ‘NOT’ are also frequently used.
Examples of Pseudo Code
Let’s consider some basic examples:
Example 1: Calculating Discount
Use variables:
price, discount, discountedPrice, of type float
Display “Enter the price of the item:”
Accept price
Display “Enter the discount percentage:”
Accept discount
discountedPrice = price - (price * discount / 100)
Display “The discounted price is $” + discountedPrice
Example 2: Selecting a Travel Destination
Use variables:
destination, cost, of type string and float
Display “Choose your travel destination:”
Display “1. Beach vacation”
Display “2. Mountain retreat”
Display “3. City tour”
Accept destination
Switch case destination:
Case destination = 1:
cost = 1000
Case destination = 2:
cost = 1200
Case destination = 3:
cost = 800
Default:
Display “Invalid choice”
Exit switch
Display “The cost for your chosen destination is $” + cost
Example 3: A survey to discover the most popular fruit.
Use variables:
apple, banana, cherry, date, fruit, of type integer
choice, of type character
apple = 0
banana = 0
cherry = 0
date = 0
Repeat
Display “Type in the letter of your favorite fruit or Q to finish:”
Display “A: Apple”
Display “B: Banana”
Display “C: Cherry”
Display “D: Date”
Display “Enter data:”
Accept choice
If choice = „A‟ then
apple = apple + 1
Else if choice = „B‟ then
banana = banana + 1
Else if choice = „C‟ then
cherry = cherry + 1
Else if choice = „D‟ then
date = date + 1
Until choice = „Q‟
Display “Apple received”, apple, “votes”
Display “Banana received”, banana, “votes”
Display “Cherry received”, cherry, “votes”
Display “Date received”, date, “votes”
Pseudo Code Guidelines
- As much as possible, use plain English.
- Avoid specific programming language syntax.
- The focus is on the logic of the solution, not the syntax of a language.
- Indentations and formatting should reflect the structure of your solution.
In a nutshell, pseudo code provides a clear, human-readable plan for your program, making it an essential tool in any developer’s toolkit. It’s your storyboard, guiding you through the process of crafting efficient and clean code. By integrating pseudo code into your routine, you’ll enhance your problem-solving skills and become more efficient as a programmer. Remember, eloquent code begins with a well-thought-out plan.
Comments